<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.targetandroid.info.alertdialog.MainActivity"> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Radio Button" android:id="@+id/btradio_aleart" android:background="@android:color/holo_orange_dark"/> <ImageButton android:layout_width="match_parent" android:layout_height="wrap_content" android:src="@android:drawable/toast_frame" android:id="@+id/btcheck_aleart" android:background="@android:color/white" /> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Exit" android:layout_gravity="bottom" android:id="@+id/btexit_aleart" android:background="@android:color/holo_green_dark"/> </LinearLayout>
3. Now Open Your MainActivity.java Add Below Code In File
package com.targetandroid.info.alertdialog; import android.app.AlertDialog; import android.content.DialogInterface; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.ImageButton; import android.widget.Toast; public class MainActivity extends AppCompatActivity { //creating variabels private Button btexit_aleart,btradio_aleart; private ImageButton btcheck_aleart; private int selected; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //Initializing views btexit_aleart=(Button)findViewById(R.id.btexit_aleart); btradio_aleart=(Button)findViewById(R.id.btradio_aleart); btcheck_aleart=(ImageButton)findViewById(R.id.btcheck_aleart); btexit_aleart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { showExitAlertDialogue(); } }); btradio_aleart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { final String[] select={"Maharashtra","Kolkata","Rajasthan","Delhi","M.p"}; AlertDialog dialog=new AlertDialog.Builder(MainActivity.this) .setTitle("Select State") .setSingleChoiceItems(select, 1, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { selected = which; } }) .setPositiveButton("Ok", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Toast.makeText(MainActivity.this, "Your State is " + select[selected], Toast.LENGTH_SHORT).show(); } }) .create(); dialog.show(); } }); btcheck_aleart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { final String[] check={"Cricket","Hockey","Football","Badminton","Volleyball","Baseball"}; AlertDialog dialog=new AlertDialog.Builder(MainActivity.this) .setTitle("Select Sports") .setMultiChoiceItems(check, null, new DialogInterface.OnMultiChoiceClickListener() { @Override public void onClick(DialogInterface dialog, int which, boolean isChecked) { int checked=which; if(isChecked){ Toast.makeText(MainActivity.this,"You Selected "+check[checked],Toast.LENGTH_SHORT ).show(); } else{ Toast.makeText(MainActivity.this,"You UnSelected "+check[checked],Toast.LENGTH_SHORT ).show(); } } }) .setPositiveButton("Ok", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { } }) .create(); dialog.show(); } }); } @Override public void onBackPressed() { showExitAlertDialogue(); } //create aleart dialogue to confirm exit of user public void showExitAlertDialogue(){ AlertDialog dialog=new AlertDialog.Builder(MainActivity.this) .setTitle("Confirm") .setMessage("Do You Really want to Exit?") .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { finish(); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { } }) .setCancelable(true) .create(); dialog.show(); } }
4. Now finally run Your Project
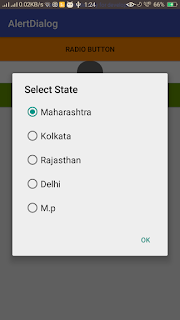
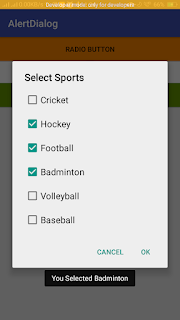
Thank You
0 comments:
Post a Comment